一、依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-mongodb</artifactId>
</dependency>
二、启动类
@SpringBootApplication(exclude = DataSourceAutoConfiguration.class)
public class MongodbdemoApplication {
public static void main(String[] args) {
SpringApplication.run(MongodbdemoApplication.class, args);
}
}
三、yml配置文件
spring:
data:
mongodb:
auto-index-creation: true
# 连接副本集,slaveOk=true 表示开启副本节点的读支持,可实现读写分离,connect=replicaSet 表示自动到副本集中选择读写的主机,replicaSet=myrs 用来指定副本集的名称
uri: mongodb://localhost:27017/数据库名
四、实体类
1、LoginInfo
@Document("表名")
public class LoginInfo {
private UserInfo userInfo;
private String id;
@Field("type_")
private String type;
private String createTime;
- 如果字段名称与数据库不符合需要加@Field(“数字库字段名”)
- 如果数据库id字段名名称为id或_id,可以不用加@Id
2、UserInfo
@Document
public class UserInfo {
private String name;
private String realname;
}
五、持久层
@Repository
public interface LoginInfoDao extends MongoRepository<LoginInfo, String> {
}
六、业务逻辑层(增删改查)
- 可以直接使用Jpa,也可以使用MongoTemplate ,以下介绍以MongoTemplate 为主
@Service
public class LoginService {
@Autowired
private MongoTemplate mongoTemplate;
@Autowired
private LoginInfoDao loginInfoDao;
public void queryLoginInfo() {
Criteria criteria = Criteria.where("type").is("操作日志");
Query query = Query.query(criteria);
long NumberOfElements = mongoTemplate.count(query, TLogEntry.class);
query.skip((long)2*5).limit(5);
query.with(Sort.by(Sort.Order.desc("id")));
List a = mongoTemplate.find(query,LoginInfo.class);
List loginList = loginInfoDao.findAll();
Optional<LoginInfo> c = loginInfoDao.findById("0003");
}
public void updateLoginInfo(){
Criteria criteria = Criteria.where("id").is("0003");
Query query = Query.query(criteria);
Update update = Update.update("type_","操作").set("create","202211");
UpdateResult updateResult = mongoTemplate.updateFirst(query,update,LoginInfo.class);
}
public void addLoginInfo(){
LoginInfo loginInfo = new LoginInfo();
loginInfo.setId("0006");
loginInfo.setCreateTime("202211");
loginInfo.setType("操作日志");
mongoTemplate.insert(loginInfo, "表名");
mongoTemplate.insert(loginInfoList, "表名");
}
public void deleteLoginInfo(){
Criteria criteria = Criteria.where("_id").is("111");
Query query = Query.query(criteria);
DeleteResult deleteResult = mongoTemplate.remove(query, LoginInfo.class);
}
}

- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
七、复杂查询(待续…)
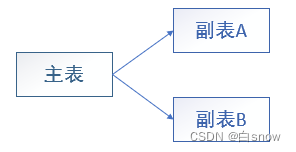
1.两表联查
LookupOperation dataAndFlow = LookupOperation.newLookup()
.from("xxx")
.localField("xxx")
.foreignField("xxx")
.as("xxx");
Criteria criteria = new Criteria();
criteria.and("xxx").gte(start).lte(end);
Aggregation aggregation = Aggregation.newAggregation(dataAndFlow, Aggregation.match(criteria), Aggregation.skip((long) (pagingPage - 1) * pagingNumberPer),
Aggregation.sort(Sort.Direction.DESC, "createTime"),
Aggregation.limit(pagingNumberPer));
AggregationResults<JSONObject> jsonObjectResult = mongoTemplate.aggregate(aggregation, "主表", JSONObject.class);
2.三表联查

- 此种情况需要建立一张公共表
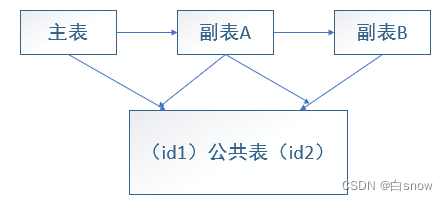
3.数组格式查询
Query query = new Query();
String keyword = result.get("value");
Pattern pattern = Pattern.compile(keyword, Pattern.CASE_INSENSITIVE);
String name = "列名." + result.get("place");
query.addCriteria(Criteria.where(name).regex(pattern));
4.时间范围查询
Criteria criteriaStart = Criteria.where("UpdateTime").gte(start).lte(end);
query.addCriteria(criteriaStart);
5.多排序查询
String[] splitSortBy = queryMap.get("sortBy").toString().split(",");
String[] splitSortDesc = queryMap.get("sortDesc").toString().split(",");
for (int i = 0; i < splitSortBy.length; i++) {
splitSortBy[i] = splitSortBy[i].replaceAll("[\\[\\] ]", "");
splitSortDesc[i] = splitSortDesc[i].replaceAll("[\\[\\] ]", "");
}
for (int i = 0; i < splitSortBy.length; i++) {
Order order = Order.by(splitSortBy[i]);
orderList.add(order);
if ("true".equals(splitSortDesc[i])) {
Order orderSort = Order.desc(splitSortBy[i]);
orderList.add(orderSort);
} else {
Order orderSort = Order.asc(splitSortBy[i]);
orderList.add(orderSort);
}
}
query.with(Sort.by(orderList));
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
八、Query、Criteria和Sort
Query:
方法 | 简介 |
---|
query(CriteriaDefinition criteriaDefinition) | 静态方法通过注入一个 CriteriaDefinition 条件对象获得Query查询对象。例如:mongoTemplate.find(Query.query(Criteria.where(“字段”).lt(“xxx”))) |
addCriteria(CriteriaDefinition criteriaDefinition) | 添加一个CriteriaDefinition 查询条件类到本次的查询 |
skip(long skip) | 跳过文档的数量,可以与limit配合使用实现分页效果 |
limit(int limit) | 查询返回的文档数量 |
with(Sort sort) | 添加一个Sort排序对象 |
with(Pageable pageable) | 添加一个Pageable分页对象。Pageable可以注入一个Sort,因此可以一起添加分页和排序。例如: Pageable pageable = new PageRequest(1,5,sort) |
Criteria:相当于SQL的where
方法 | 简介 |
---|
where(String key) | 自定义查询条件mongoTemplate.find(Query.query(Criteria.where(“字段”).lt(“xxx”))) |
and(String key) | 与操作 |
gt(Object o) | 大于 |
gte(Object o) | 大于等于 |
in(Object… o) | 包含 |
is(Object o) | 等于 |
lt(Object o) | 小于 |
lte(Object o) | 小于等于 |
not() | 非 |
regex(String re) | 正则表达式 |
andOperator(Criteria… criteria) | 创建与操作 |
orOperator(Criteria… criteria) | 创建或操作 |
Sort:创建查询排序
方法 | 简介 |
---|
Sort(Sort.Direction direction, String… properties) | 构造方法创建一个排序。direction为排序方向的枚举类型,properties为排序字段数组 |
and(Sort sort) | 多个排序条件连接 |
ascending() | 返回升序排列对象 |
descending() | 返回降序排列对象 |
九、索引创建
索引建立
十、使用GridFS存储大数据
GridFS
十一、注意事项
- 宁可冗余一些数据,也尽量避免连表查询(连表效率真的很低而且副表排序也是问题)。
- 单个文件存储不要过大,超过16MB时会报错。
- 可以通过调整大小解决(不推荐,因为会影响查询效率)。
- 可以把大内容存到本地文件,然后存路径到数据库。
- 可以使用GridFS
- 排序不要过多,因为排序的时候会加载内容到内存进行排序,大小限制为32MB,超过会报错。