问题
1 C++中多个线程共同执行一个实例函数,该函数是在线程的栈空间吗?对于函数中的多线程共享变量又是存储在哪里呢?
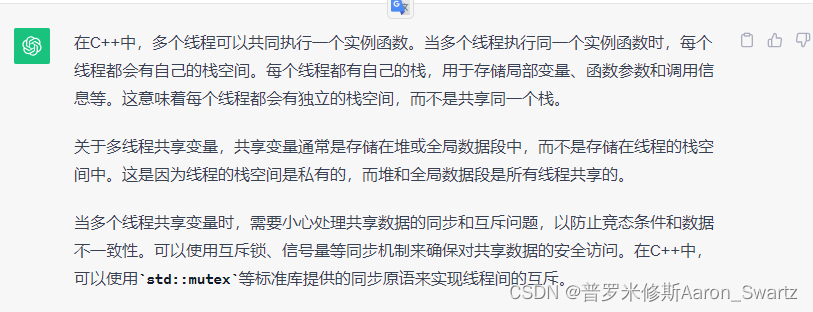
example: 在该例子中线程绑定当前对象(this)的实例函数captureVideo, 并将int参数传递过去。但是对于captureVideo中多个线程共享访问的变量,必须通过锁来处理,以防止出现竞态条件
void LoopHTCVPort::KeepCapture()
{
std::thread t0(std::bind(&LoopHTCVPort::captureVideo, this, 0));
t0.detach();
std::thread t1(std::bind(&LoopHTCVPort::captureVideo1, this, 1));
t1.detach();
std::thread t2(std::bind(&LoopHTCVPort::captureVideo2, this, 2));
t2.detach();
std::thread t3(std::bind(&LoopHTCVPort::captureVideo3, this, 3));
t3.detach();
std::thread t4(std::bind(&LoopHTCVPort::captureVideo4, this, 4));
t4.detach();
}
void LoopHTCVPort::captureVideo(int cameraId)
{
RobotModel& model = RobotModel::GetGlobalInstance();
int fps = 20;
int delay = 1000 / fps;
tSdkFrameHead frameinfo;
BYTE* pbyBuffer;
unsigned char* g_pRgbBuffer;
int iDisplayFrames = 0;
int width = 1920;
int height = 1080;
std::time_t last = ::GetTimeStamp();
cv::Mat tempImage;
while (true)
{
int distance = model.Odometer.GetRealValue();
try
{
int index = 0;
int hCamera;
for(auto iter = g_hCameraArray.begin(); iter != g_hCameraArray.end(); iter++)
{
if (cameraId != index)
{
continue;
}
std::time_t now = ::GetTimeStamp();
cout << "capture last: " << (now - last) << endl;
last = ::GetTimeStamp();
g_pRgbBuffer = (unsigned char*) malloc (g_tCapabilityArray[cameraId].sResolutionRange.iHeightMax * g_tCapabilityArray[cameraId].sResolutionRange.iWidthMax * 3);
hCamera = *iter;
int cplaystatus = CameraPlay(hCamera);
if(cplaystatus != CAMERA_STATUS_SUCCESS)
{
cout << "CameraPlay faliure! cplaystatus"<< cplaystatus << endl;
}
int cgimagestatus = CameraGetImageBuffer(hCamera, &frameinfo, &pbyBuffer, 1000);
if(cgimagestatus == CAMERA_STATUS_SUCCESS)
{
int processstatus = CameraImageProcess(hCamera, pbyBuffer, g_pRgbBuffer, &frameinfo);
if(processstatus != CAMERA_STATUS_SUCCESS)
{
cout << "CameraImageProcess error! code: " << processstatus << endl;
}
tempImage = cv::Mat(cv::Size(frameinfo.iWidth, frameinfo.iHeight), frameinfo.uiMediaType == CAMERA_MEDIA_TYPE_MONO8 ? CV_8UC1 : CV_8UC3, g_pRgbBuffer);
int releasestatus = CameraReleaseImageBuffer(hCamera, pbyBuffer);
if(releasestatus != CAMERA_STATUS_SUCCESS)
{
cout << "CameraReleaseImageBuffer error! code: " << releasestatus << endl;
}
funcallback(cameraId, tempImage);
} else
{
cout << "CameraGetImageBuffer occur error! code: " << cgimagestatus << endl;
}
free(g_pRgbBuffer);
index ++;
break;
}
}
catch(std::exception e)
{
cout << "Detection camera capture pictures error! " << e.what() << endl;
releaseHTCamera();
free(g_pRgbBuffer);
}
}
}

- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 67
- 68
- 69
- 70
- 71
- 72
- 73
- 74
- 75
- 76
- 77
- 78
- 79
- 80
- 81
- 82
- 83
- 84
- 85
- 86
- 87
- 88
- 89
- 90
- 91
- 92
- 93
- 94
- 95
- 96
- 97
- 98
- 99
- 100
2 在C++中,多个线程共同执行一个实例函数和共同执行一个静态函数有一些关键的区别。以下是一些主要的区别?
