Vue3-ref函数、reactive函数的响应式
在这之前,先讲Vue2的响应式处理
- Vue2原本使用的是
Object.defineProperty
的响应式处理方式
- methods方法中的this.name指的是vm.name
- return的name属性在通过this.name的间接调用时,通过了
Object.defineProperty
响应式处理
<template>
<h2>姓名:{{name}}</h2>
<button @click="modifyName">修改姓名</button>
</template>
<script>
export default {
name : 'App',
data(){
return {
name : '张三'
}
},
methods:{
modifyName(){
this.name = '李四'
}
}
}
</script>
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
ref函数的响应式(适用于基本数据类型)
- 原理:
- ref函数通过对数据进行一个包装,然后返回一个全新的对象,叫做引用对象RefImpl
- 在这个RefImpl对象中有一个value属性,而value属性底层调用了
Object.defineProperty
响应式 - 而value属性有set和get:
- 读取RefImpl对象的value属性时:get
- 修改RefImpl对象的value属性时:set
- 使用前导入:
import {ref} from 'vue'
基本数据类型的响应式处理 ref(基本数据类型)
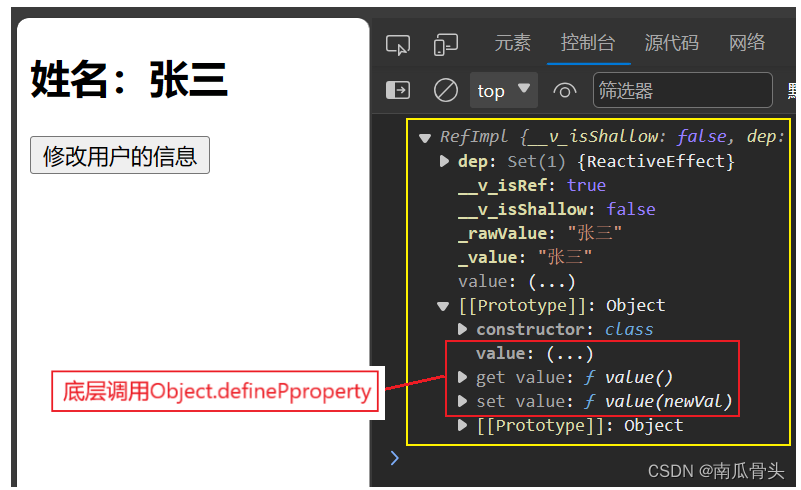
<template>
<h2>姓名:{{nameRefImpl}}</h2>
<button @click="modifyInfo">修改用户的信息</button>
</template>
<script>
import {ref} from 'vue'
export default {
name : 'App',
setup(){
let nameRefImpl = ref('张三')
console.log(nameRefImpl.value);
function modifyInfo(){
nameRefImpl.value = '李四'
}
return{nameRefImpl, modifyInfo}
}
}
</script>
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
对象形式的响应式处理 ref({})(不用)
- 用ref函数包装对象形式,底层也就是reactive函数的调用
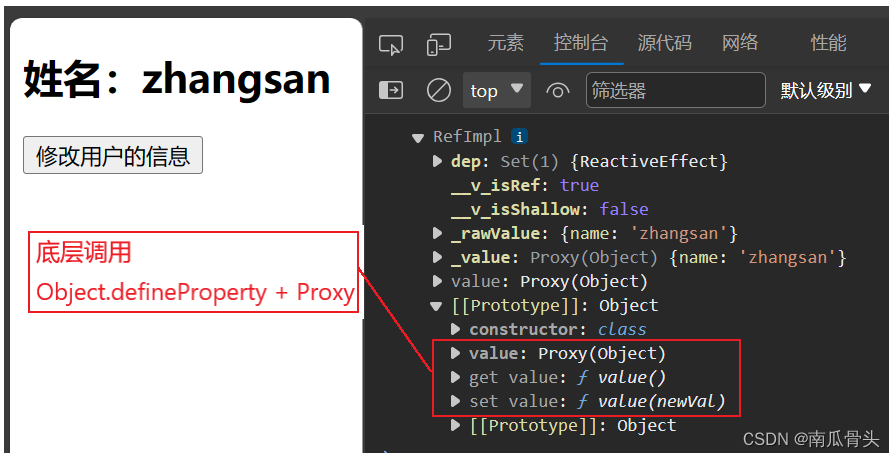
<template>
<h2>姓名:{{nameRefImpl.name}}</h2>
<button @click="modifyInfo">修改用户的信息</button>
</template>
<script>
import {ref} from 'vue'
export default {
name : 'App',
setup(){
let nameRefImpl = ref({
name : 'zhangsan'
})
function modifyInfo(){
nameRefImpl.value.name = 'lisi'
}
return{nameRefImpl, modifyInfo}
}
}
</script>
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
reactive函数的响应式(适用于对象形式)
- 原理:将一个对象直接包裹,实现响应式,底层生成一个Proxy对象
- 使用前导入:
import { reactive } from 'vue'
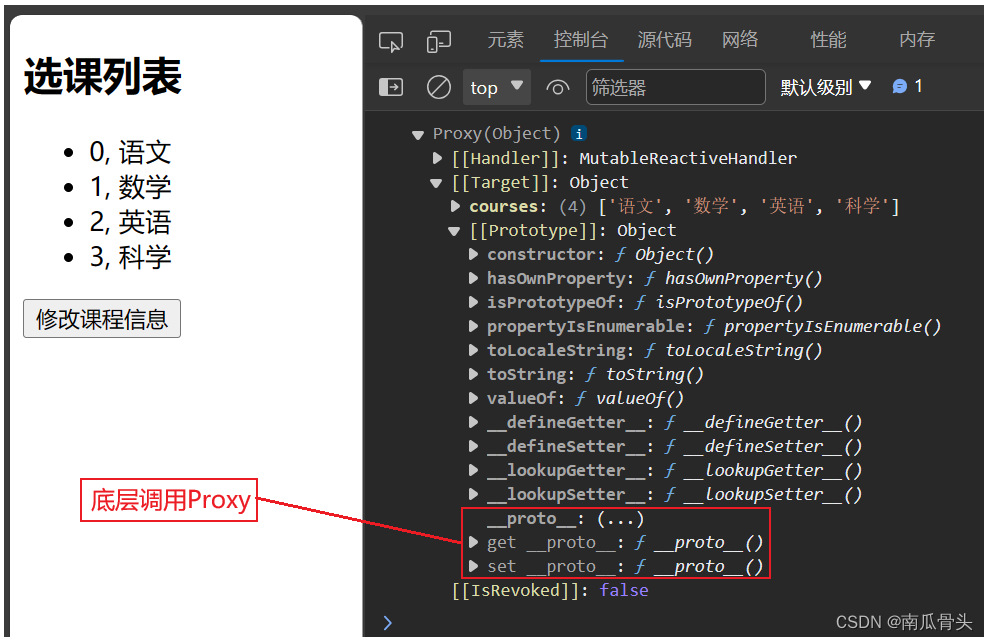
<template>
<h2>选课列表</h2>
<ul>
<li v-for="(course, index) in courseProxy.courses" :key="index">
{{index}}, {{course}}
</li>
</ul>
<button @click="modifyCourse">修改课程信息</button>
</template>
<script>
import { reactive } from 'vue'
export default {
name : 'App',
setup() {
let courseProxy = reactive({
courses : ['语文', '数学', '英语', '科学']
})
function modifyCourse(){
userProxy.courses[2] = '地理'
}
return{courseProxy, modifyCourse}
}
}
</script>
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30